8.2 Project 2: Data Analysis – Analyzing a Dataset with Pandas and Matplotlib
Data analysis is a crucial process for extracting knowledge and insights from data. By utilizing Python libraries like Pandas and Matplotlib, you can effectively analyze datasets, visualize data relationships, and communicate findings effectively.
8.1 Project 1: Web Scraper
Project 1: Web Scraper Objectives: Introduction: Web scraping is the process of extracting data from websites. It involves sending HTTP requests to retrieve HTML content and then parsing the HTML to extract the desired information. Python provides powerful libraries for web scraping, making it a convenient tool for data collection from the web. Step 1: […]
7.2 Decorators and Generators – Understanding Decorators, Working with Generators
Welcome to an exciting lesson where we’ll explore two powerful features in Python: Decorators and Generators. Decorators provide a clean and efficient way to modify or extend the behavior of functions, while Generators offer a memory-efficient mechanism for creating iterators. In this lesson, we’ll dive deep into the concepts of decorators, understand their syntax and […]
7.1 Exception Handling
Objectives: Introduction: Exceptions represent unexpected events that occur during program execution, potentially disrupting the normal flow of the program. Java provides a comprehensive exception handling mechanism to gracefully handle and recover from such errors. Types of Exceptions: Java categorizes exceptions into two main types: Try-Catch Blocks: The try-catch block is the fundamental mechanism for exception […]
6.2 CSV and JSON file operations
Objectives: Introduction: CSV (Comma Separated Values) and JSON (JavaScript Object Notation) are widely used file formats for storing and exchanging data. CSV files store data in a tabular format, separated by commas, while JSON files store data in a key-value format. Java provides libraries for handling both CSV and JSON files, enabling you to read, […]
6.1: Reading and Writing Files
Objectives: Introduction: Files are essential components of software development, allowing for the storage and retrieval of data. Java provides the java.io package for file handling, including classes for creating, opening, and closing files, reading and writing data, and managing file permissions. Text Files and Binary Files: Files can be classified as text files or binary […]
5.2 Inheritance and Polymorphism – Creating Subclasses, Implementing Polymorphism
Welcome to an enriching lesson where we’ll delve into the powerful concepts of Inheritance and Polymorphism in Python. Inheritance allows us to create a new class based on an existing one, promoting code reuse and extensibility. Polymorphism, on the other hand, empowers objects to take on multiple forms, enhancing flexibility and readability. In this lesson, […]
5.1 Introduction to OOP – Basics of Object-Oriented Programming, Classes, and Objects
Welcome to the fundamental concepts of Object-Oriented Programming (OOP) in Python. Object-Oriented Programming is a paradigm that enables us to model real-world entities using objects, combining data and functionality in a structured and modular way. In this lesson, we’ll explore the core principles of OOP, understand classes and objects, and learn how to implement them in Python.
4.3 Sets – Introduction to Sets and Set Operations
Welcome to this lesson on Python sets! We will explore the concept of sets in Python and learn about various set operations. Sets are an essential data structure in Python that allow us to store unique and unordered elements. They can be incredibly useful for tasks such as removing duplicates from a list, performing mathematical operations, and more. Learn about adding and removing elements from sets, set union, intersection, difference, and symmetric difference in Python.
4.2 Dictionaries – Understanding Dictionaries, Dictionary Operations
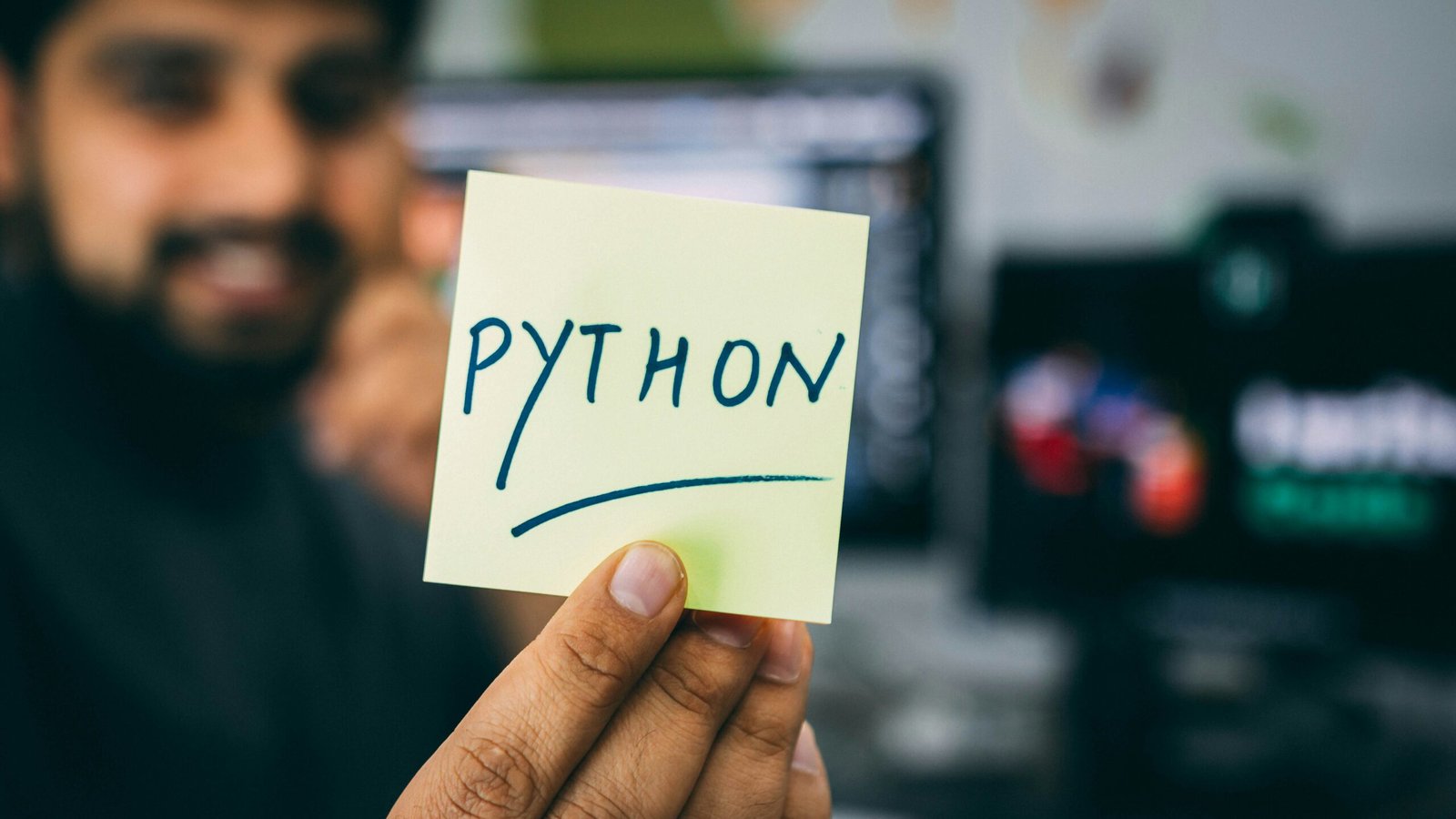
In Python, a dictionary is a collection of key-value pairs. It is an unordered, mutable, and iterable data structure. Dictionaries are commonly used to store and retrieve data based on a unique key.